Prototype Pattern
Prototype pattern is used for cloning purpose. Specially when creating new object requires intensive work like a lot of queries etc. then instead of doing that, cloning is done by using Prototype Pattern.
Prototype Pattern Example
Here, I have given example of 2 animals Deer and Horse.
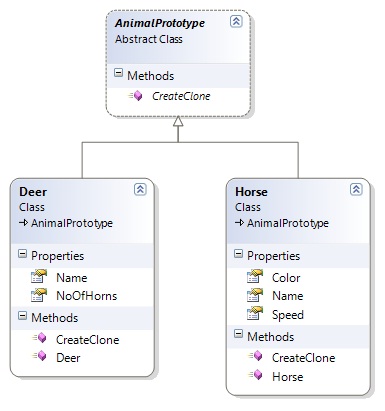
public abstract class AnimalPrototype { abstract public AnimalPrototype CreateClone(); } public class Deer : AnimalPrototype { public string Name { get; set; } public int NoOfHorns { get; set; } public Deer(string name, int noOfHorns) { this.Name = name; this.NoOfHorns = noOfHorns; } // Shallow copy public override AnimalPrototype CreateClone() { return (AnimalPrototype)this.MemberwiseClone(); } } public class Horse : AnimalPrototype { public string Name { get; set; } public int Speed { get; set; } public string Color { get; set; } public Horse(string name, int speed,string color) { this.Name = name; this.Speed = speed; this.Color = color; } // Shallow copy public override AnimalPrototype CreateClone() { return (AnimalPrototype)this.MemberwiseClone(); } }
class Program { static void Main(string[] args) { Deer deer = new Deer("Deer-X",2); Deer deerWithOneHorn = (Deer)deer.CreateClone(); deerWithOneHorn.NoOfHorns = 1; Console.WriteLine("*** Deer with One Horn***"); Console.WriteLine("No. of Horns = "+deerWithOneHorn.NoOfHorns); Console.WriteLine("Name = " + deerWithOneHorn.Name); Horse horse = new Horse("Horse-Y",30,"Black"); Horse horseWithBrownColor = (Horse)horse.CreateClone(); horseWithBrownColor.Color = "Brown"; Console.WriteLine("*** Horse with Brown Color***"); Console.WriteLine("Color = " + horseWithBrownColor.Color); Console.WriteLine("Name = " + horseWithBrownColor.Name); Console.WriteLine("Speed = " + horseWithBrownColor.Speed); Console.ReadKey(); } }
Prototype Pattern Output
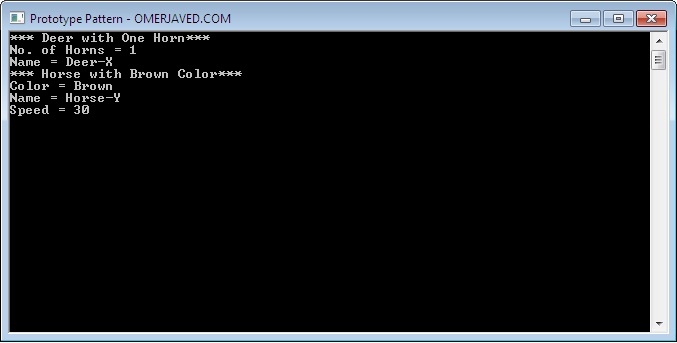