Facade Pattern
As per Facade pattern, subsystem(s) can have simplified interface. Client interacts with Facade and Facade interacts with the subsystem(s).
Facade Pattern Example
Here is an example of a Facade Pattern with three subsystems.
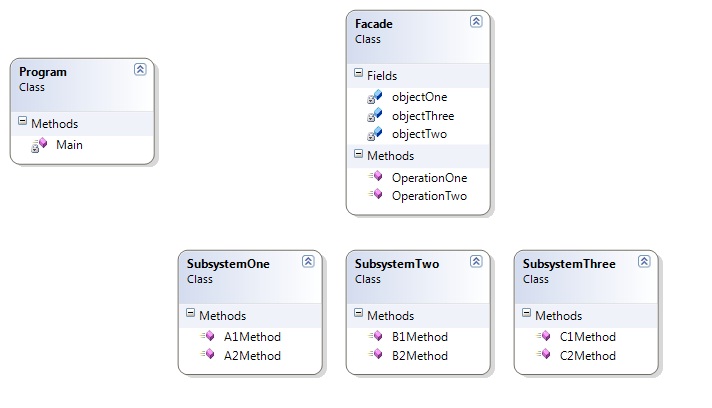
class SubsystemOne { public void A1Method() { Console.WriteLine("OP-A1 of SubsystemOne is called."); } public void A2Method() { Console.WriteLine("OP-A2 of SubsystemOne is called."); } } class SubsystemTwo { public void B1Method() { Console.WriteLine("OP-B1 of SubsystemTwo is called."); } public void B2Method() { Console.WriteLine("OP-B2 of SubsystemTwo is called."); } } class SubsystemThree { public void C1Method() { Console.WriteLine("OP-C1 of SubsystemThree is called."); } public void C2Method() { Console.WriteLine("OP-C2 of SubsystemThree is called."); } } public class Facade { SubsystemOne objectOne = new SubsystemOne(); SubsystemTwo objectTwo = new SubsystemTwo(); SubsystemThree objectThree = new SubsystemThree(); public void OperationOne() { Console.WriteLine("*** Operation One ***"); objectOne.A1Method(); objectOne.A2Method(); objectTwo.B1Method(); } public void OperationTwo() { Console.WriteLine("*** Operation Two ***"); objectTwo.B2Method(); objectThree.C1Method(); objectThree.C2Method(); } }
class Program { static void Main(string[] args) { Facade facade = new Facade(); facade.OperationOne(); Console.ReadKey(); } }
Facade Pattern Output
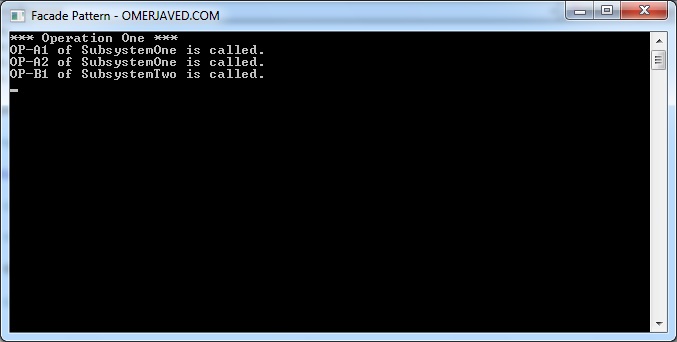