Strategy Pattern
Strategy pattern says that we should not use inheritance for inheriting different behavior but instead of it we should use separate interfaces for it and encapsulate the behavior. There should be decoupling between the behavior and the class that uses this behavior. By having this, we come up with such design where changing in behavior class, does not break the classes which are using it. This pattern applies for cases when classes differ only in behavior. If we do not implement strategy pattern then we would have to add a lot of conditions for each case in a single method. While using this pattern, different classes implement the interface for behavior.
Strategy Pattern Example
Here is an example of School system. There are many types of employees there and each have different role. For example, Teacher is there to teach the students. Course Advisor has the task to give advices to student regarding their course. Moreover, administrator is there in school to perform administrative tasks.
Although we could add one method performTask() in Employee class and inherent it in the three different subclasses. But here comes the dependency problem. In performTask(), there would be conditions for each type of child object of Employee. If we would want to make any change in future then many classes would need to be changed and recompiled.
So solution is Strategy pattern. By applying it, we would make an interface of IDutyBehavior. In addition to it, we would implement this behavior (interface) in the respective child classes. For example, Teaching is implementing IDutyBehavior interface.
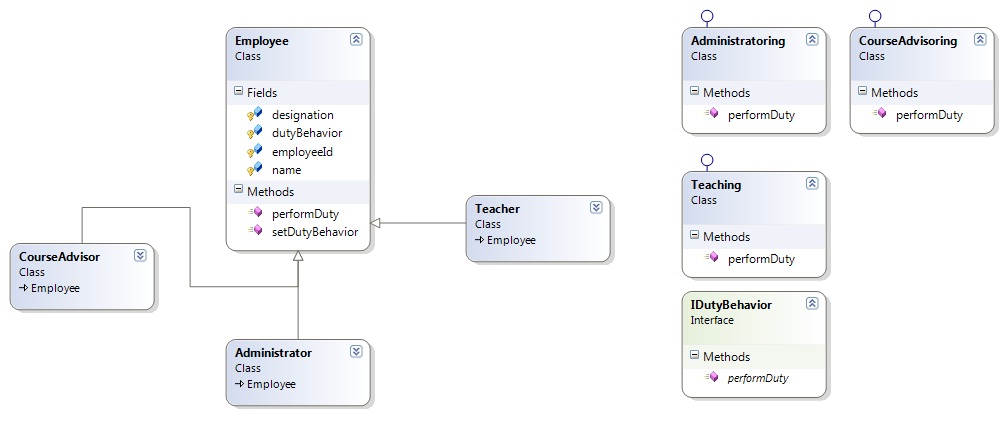
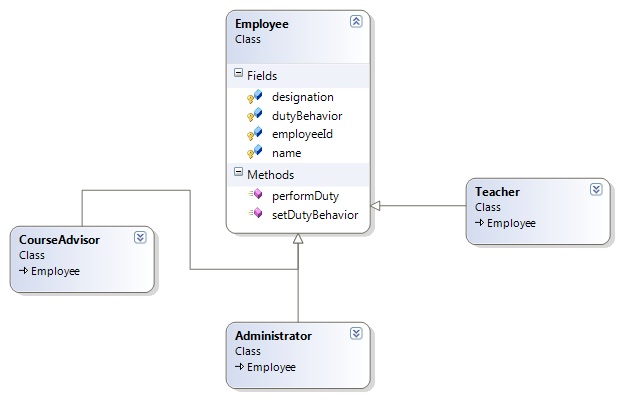
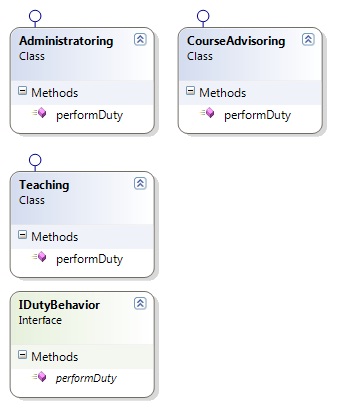
public interface IDutyBehavior { string performDuty(); } public class Teaching : IDutyBehavior { public string performDuty() { // teach return "teaching"; } } public class CourseAdvisoring : IDutyBehavior { public string performDuty() { // Give advices on the course return "Course Advices"; } } public class Administratoring : IDutyBehavior { public string performDuty() { // Perform administration tasks return "Administering"; } }
public class Employee { protected IDutyBehavior dutyBehavior; protected int employeeId; protected string name; protected string designation; public void setDutyBehavior(IDutyBehavior dutyBehavior) { this.dutyBehavior = dutyBehavior; } public string performDuty() { if(this.dutyBehavior!=null) { return this.dutyBehavior.performDuty(); } return string.Empty; } } public class Teacher : Employee { public Teacher() { setDutyBehavior(new Teaching()); } } public class CourseAdvisor : Employee { public CourseAdvisor() { setDutyBehavior(new CourseAdvisoring()); } } public class Administrator : Employee { public Administrator() { setDutyBehavior(new Administratoring()); } }
static void Main(string[] args) { Teacher teacher = new Teacher(); string action = teacher.performDuty(); Console.WriteLine(action); Console.ReadKey(); //////////////////////////// CourseAdvisor cAdvisor = new CourseAdvisor(); action = cAdvisor.performDuty(); Console.WriteLine(action); Console.ReadKey(); ///////////////////// Administrator admin = new Administrator(); action = admin.performDuty(); Console.WriteLine(action); Console.ReadKey(); }