Factory Method
Factory Method gives the flexibility that it provides an interface for the creation of the objects and its gives the responsibility of deciding which class to instantiate to subclasses.
Factory Method Example
Here is an example of a Shoe-Store. Shoe-Store has a factory method CreateShoe(…) which returns IShoe. Subclass RegionStyleShoe, depending on the type parameter sent to CreateShoe() method decides which instance of Shoe should be created.
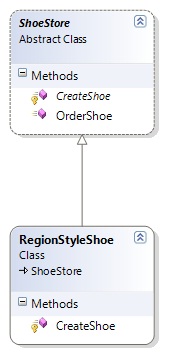
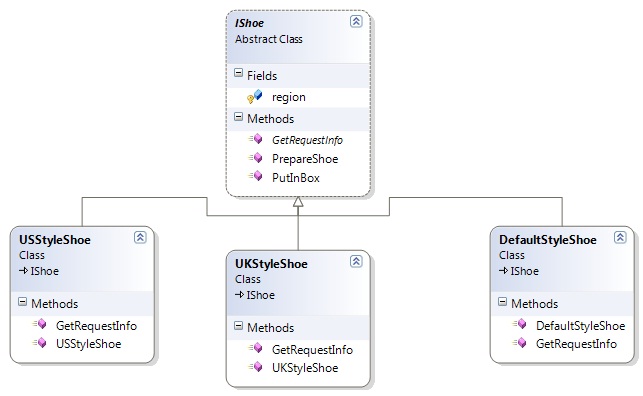
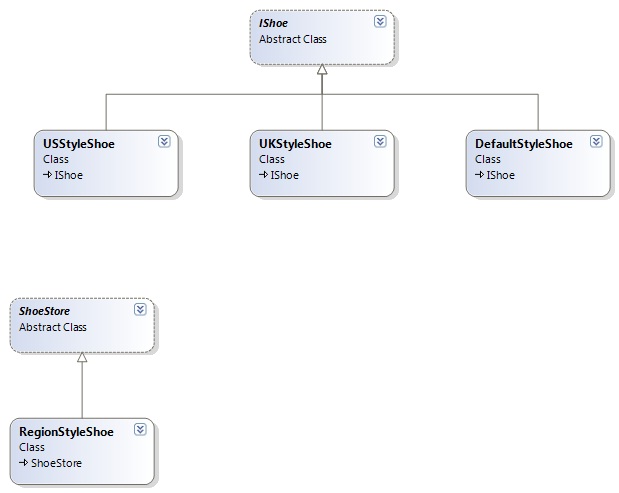
// Creator class (abstract class) public abstract class ShoeStore { public void OrderShoe(string type) { IShoe shoe = CreateShoe(type); string rqtInfo = shoe.GetRequestInfo(); Console.WriteLine(rqtInfo); // Preparing shoe.PrepareShoe(); // Boxing shoe.PutInBox(); } // Factory Method protected abstract IShoe CreateShoe(string type); } public class RegionStyleShoe : ShoeStore { protected override IShoe CreateShoe(string type) { IShoe shoe = null; switch (type.ToLower()) { case "us": shoe = new USStyleShoe(); break; case "uk": shoe = new USStyleShoe(); break; default: shoe = new DefaultStyleShoe(); break; } return shoe; } }
public abstract class IShoe //Product abstract class { protected string region = string.Empty; public abstract string GetRequestInfo(); public void PrepareShoe() { Console.WriteLine("Preparing "+this.region+" Shoes!"); } public void PutInBox() // For automatic handling of putting Shoes in Box { Console.WriteLine("Boxing " + this.region + " Shoes!"); } } public class USStyleShoe : IShoe { public USStyleShoe() { this.region = "US"; } public override string GetRequestInfo() { return "Shoe Request has come at United States branch"; } } public class UKStyleShoe : IShoe { public UKStyleShoe() { this.region = "UK"; } public override string GetRequestInfo() { return "Shoe Request has come at United Kingdom branch"; } } public class DefaultStyleShoe : IShoe { public DefaultStyleShoe() { this.region = "Default"; } public override string GetRequestInfo() { return "Default Styled Shoes (from US)"; } }
namespace FactoryMethodPattern { class Program { static void Main(string[] args) { RegionStyleShoe rgnShoe = new RegionStyleShoe(); rgnShoe.OrderShoe("us"); Console.ReadKey(); } } }