Adapter Pattern
According to Adapter pattern, we can convert the interface of class to another interface as per client requirement.
Adapter Pattern Example
Here is an example of a Laptop which has Two-pin Plug but you want to plug it into Three-point switch.
So solution is Adapter Pattern. By applying this pattern, we will plug our two-pin plug into 2-pin to 3-pin converter (adapter) and then plug it into 3-point switch.
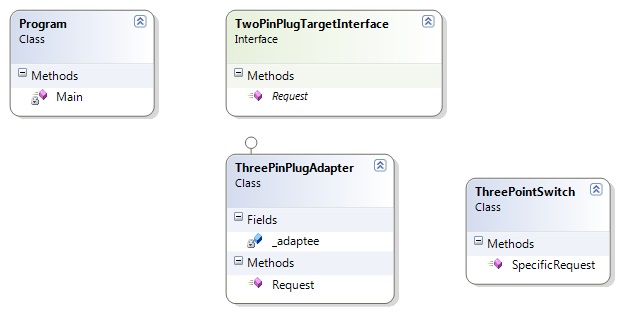
// "Target" interface interface TwoPinPlugTargetInterface { void Request(); } // "Adapter" class class ThreePinPlugAdapter : TwoPinPlugTargetInterface { private ThreePointSwitch _adaptee = new ThreePointSwitch(); public void Request() { _adaptee.SpecificRequest(); } } // "Adaptee" class class ThreePointSwitch { public void SpecificRequest() { Console.WriteLine("SpecificRequest() method of "+ "ThreePointSwitch(Adaptee)"); } }
class Program { static void Main(string[] args) { TwoPinPlugTargetInterface target = new ThreePinPlugAdapter(); target.Request(); Console.ReadKey(); } }